Are you a C# developer looking to enhance user interaction in your applications by efficiently handling the SelectedIndexChanged event? In this comprehensive guide, we will walk you through the step-by-step process of calling the SelectedIndexChanged event in C#. This event is vital for dynamically updating elements based on user selections in controls like dropdown lists or list boxes.
Understanding how to call the SelectedIndexChanged event properly can greatly improve the usability and functionality of your C# applications. We will cover the necessary syntax, best practices, and potential pitfalls to avoid when working with this event. By the end of this guide, you will have the confidence to implement and harness the power of the SelectedIndexChanged event in your C# projects.
Understanding the SelectedIndexChanged Event in C#
The SelectedIndexChanged event in C# is a crucial event that is commonly used in user interface programming, especially when working with dropdown lists or combo boxes. This event is triggered whenever the selected index of the control is changed, allowing you to perform specific actions based on the user’s selection.
Working Mechanism of SelectedIndexChanged Event
When a user interacts with a dropdown list or a combo box in a C# application and changes the selected item, the SelectedIndexChanged event is raised. This event provides you with the opportunity to dynamically respond to the user’s selection.
You can handle this event by associating a method with the control’s SelectedIndexChanged event handler. This method will be executed whenever the selected index changes, allowing you to update the application’s behavior accordingly.
Implementing the SelectedIndexChanged Event
To implement the SelectedIndexChanged event in C#, you need to first identify the dropdown list or combo box control you want to monitor for changes. Then, you can add the event handler function to the control’s SelectedIndexChanged event.
Here is a simple example of how to add the event handler for a dropdown list:
- Create a new Windows Forms Application in Visual Studio.
- Drag and drop a ComboBox control onto the form.
- Double-click on the ComboBox control to generate the event handler method.
- Write your custom logic inside the event handler to handle the selected index change event.
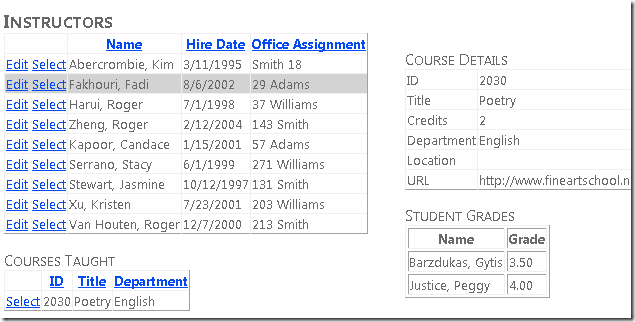
Creating the Event Handler Method
In C#, to call the SelectedIndexChanged event, you first need to create the event handler method. This method will be triggered whenever the selected index of a dropdown list or combo box changes.
Step 1: Define the Event Handler
To create the event handler, you can use the following syntax:
private void YourComboBox_SelectedIndexChanged(object sender, EventArgs e) { // Your code logic here }
Step 2: Wire Up the Event Handler
After defining the event handler, you need to wire it up to the SelectedIndexChanged event of the specific dropdown list or combo box. You can do this by using the following code:
YourComboBox.SelectedIndexChanged += new EventHandler(YourComboBox_SelectedIndexChanged);
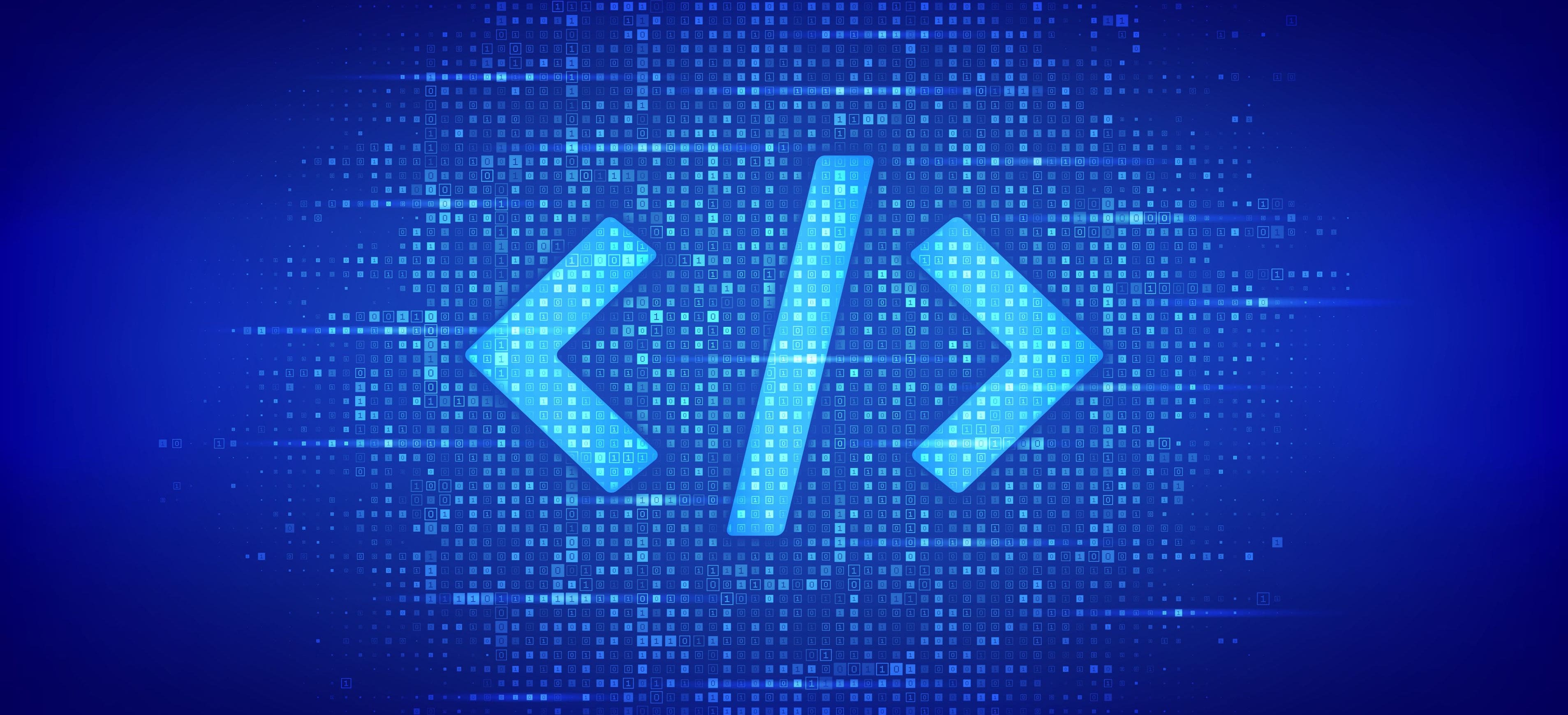
Attaching the Event Handler to the Control
When working with C# and handling events such as the SelectedIndexChanged event, it is crucial to know how to attach the event handler to the control properly. This ensures that the desired function is executed when the event is triggered.
Step 1: Find the Control
First, you need to identify the control for which you want to handle the SelectedIndexChanged event. This could be a ComboBox, ListBox, or any other control that supports this event.
Step 2: Add the Event Handler
Once you have identified the control, you can add the event handler by using the following syntax:
control.SelectedIndexChanged += new EventHandler(YourSelectedIndexChangedMethod);
Step 3: Implement the Event Handler
Now, you need to create the method that will handle the SelectedIndexChanged event. This method should match the signature of the EventHandler delegate.
Step 4: Write the Event Handling Code
Inside the event handler method, write the code that should be executed when the SelectedIndexChanged event is triggered. This could include updating UI elements, processing data, or any other desired action.
Implementing Custom Logic in the Event Handler
When working with C# and the SelectedIndexChanged event, you may sometimes need to implement custom logic within the event handler to cater to specific requirements. To do this effectively, you can follow the steps outlined below to ensure smooth handling of the event and seamless execution of your custom code.
Step 1: Accessing the Event Handler
Begin by accessing the SelectedIndexChanged event handler of the desired control, whether it’s a DropDownList or other relevant control. This is where you will be adding your custom logic to respond to changes in the selected index.
Step 2: Implementing Custom Logic
Within the event handler, you can write your custom code to handle the selected index change event. This code can include a variety of actions, such as updating other controls on the form, fetching data from a database based on the new selection, or triggering additional events.
You can use conditional statements and looping structures to tailor the behavior of your application based on the selected index. Ensure that your custom logic is efficient and error-free to maintain the stability of your application.
Testing and Troubleshooting the Event
After successfully implementing the SelectedIndexChanged event in your C# application, it is crucial to test and troubleshoot it to ensure seamless functionality. Proper testing can help identify any potential issues and ensure that the event behaves as intended.
1. Testing Functionality
One of the initial steps in testing the SelectedIndexChanged event is to simulate user interaction with the control that triggers the event. This can involve selecting different options in a dropdown list or changing the index of a tab control to verify if the event is fired accurately.
2. Debugging Process
When troubleshooting the event, debugging tools provided by Visual Studio can be immensely helpful. Setting breakpoints within the event handler allows you to inspect variables, track flow, and identify errors in the event logic.
Frequently Asked Questions
-
- What is the SelectedIndexChanged event in C#?
- The SelectedIndexChanged event in C# is an event that is raised when the selected index of a DropDownList, ComboBox, ListBox, or similar control is changed.
-
- How can I call the SelectedIndexChanged event in C#?
- To call the SelectedIndexChanged event in C#, you can directly handle the event in your code or you can trigger the event programmatically using the event handler.
-
- Can you provide a step-by-step guide on how to call the SelectedIndexChanged event in C#?
- Yes, in the blog post we have provided a detailed step-by-step guide on how to call the SelectedIndexChanged event in C# with code examples and explanations.
-
- What controls does the SelectedIndexChanged event work with in C#?
- The SelectedIndexChanged event works with controls like DropDownList, ComboBox, ListBox, and similar controls in C#.
Final Thoughts
Mastering how to call the SelectedIndexChanged event in C# is essential for enhancing the functionality of your applications. By following the step-by-step guide outlined in this blog, you can leverage this event effectively to trigger specific actions based on user selections.
Remember, understanding the intricacies of event handling in C# is crucial for developing responsive and interactive software. Whether you are a beginner or an experienced developer, knowing how to implement the SelectedIndexChanged event will undoubtedly elevate your coding skills.
So, next time you encounter a scenario where you need to respond to changes in a selection control, refer back to this guide to confidently call the SelectedIndexChanged event in C#.